Building a music app Part 4: Creating the Frond-end with NextJs and Tailwind CSS.
Building a music app Frond-end with NextJs and Tailwind CSS
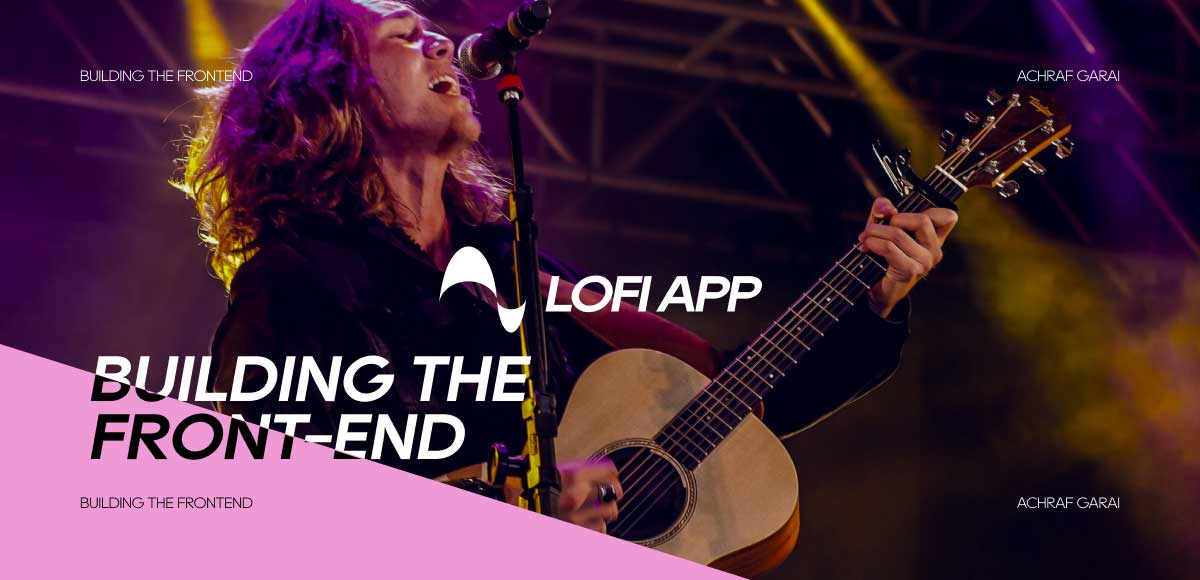
After deciding to move forward with the most recent prototype in Figma, I had already a clear vision of what I was trying to build. And, I also had a simple design system documented in Figma, which made building the UI a very simple process.
Component structure
I split the components into 3 main sections as follows
- Components/UI contains the UI primitives used in the design, like buttons, sliders, progress bars and the grid system.
- Components/Playlist Contains the components for a playlist like the playlist card, and the track card
- Components/Player Contains the components for the audio player components like the slider, volume controls etc
- Components/Common Containing elements like the Navigation, Footer.
I organized each of the components in its own folder that contains the following.
- .tsx for the component logic
- index.ts to export the component
- .module.css which contains the styling code.
Using Tailwind CSS
Tailwind is a CSS framework that helped me apply quick styling to the different components without needing to write long CSS code. Below is an example of tailwind used to style a simple Grid component.
Multi-themes with Next-themes
Next-themes is a library that makes working with themes very easy. I create a simple Theme selector UI component and use the hook provided by the library to switch themes.
After that I needed to create the configuration of CSS variables in the base CSS stylesheet that contains a simple configuration for each theme, outlining things like accent, text and background colors.
Audio Player with Typescript
The audio Player was the biggest challenge of this project. Luckily, I found a simple audio player implementation in typescript from an Engineering team that worked on a similar project.
The project provided a state that takes an array of tracks and provides simple controls like Play/Pause, volume controls etc.
To adapt the project to my use case, I had to add few functions to the player class like changing the playlist, and loading the tracks for each playlist.
Data fetching with NextJs
NextJs provides us with 2 great ways to fetch data from the back-end. GetServerSideProps is called on each client request and used for cases where data is changed frequently, like a news feed.
GetStaticProps is called on build time and is used to build static pages that don't change very often, like blog pages etc.
I decided to fetch data during build time because the playlist pages won't change very frequently.
Apollo Client and GraphQL
Apollo client is a makes it easier to work with GraphQL. It takes care of things like in cache memory etc. The code below show how this library is used with GetStaticProps to send a GraphQL query and load the playlist data from the server.
Result
Get the code
The full source code of the NextJs app is available here.